Javascript Binary Clock
Thursday, December 26th 2024
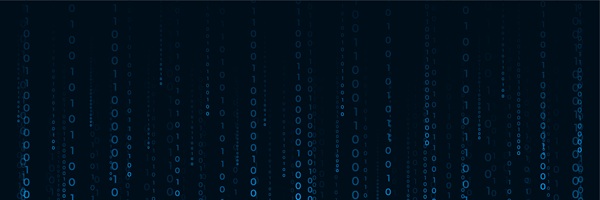
Happy Holidays everybody! Just a quick post to show off a Binary Clock I made using HTML/CSS/Javascript. This is similar to the clocks once sold by Think Geek. I always wanted one of my own, but could never justify the cost. But I figure why not try my hand at making one in software. Each LED is just a DIV element with a 100% round border with two CSS classes for each state (off/on). I wrote some code that runs every second to generate a time string. I convert the time string to binary and update the LEDs. Feel free to look at the code below the example. As always, code available at your own risk!
ClockBinary Clock
0
Categories: Programming
Tags: javascript